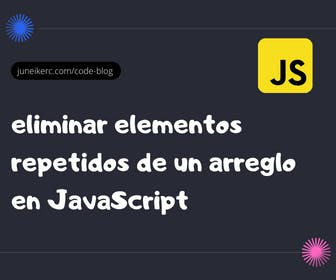
Arrays are a very useful data structure in JavaScript as they allow you to store multiple values in a single variable. However, sometimes it's necessary to remove duplicate elements from an array in order to work with the data more effectively.
In this article, I'll show you how to remove duplicate elements from an array in JavaScript in different ways.
1 - Removing Duplicate Values from an Array with .filter()
With this method, you can iterate through the array and return a new array with all the elements that meet a specific condition.
To remove duplicate elements from an array using .filter(), you should use the indexOf() function to check if the element is in the array or not. If the element is not in the array, it means it's unique and should be included in the new array.
For example:
let myArray = [1, 2, 3, 4, 5, 2, 3, 4];let arrayWithoutDuplicates = myArray.filter(function (element,index,arr) {return arr.indexOf(element) === index;});console.log(arrayWithoutDuplicates); // [1, 2, 3, 4, 5]
2 - Using the .reduce() Method
This method reduces the array to a single value by executing a function for each element.
To remove duplicate elements from an array with .reduce(), you should create a function that iterates through the array and, in the event that the element is not found in the result array, adds it.
let myArray = [1, 2, 3, 4, 5, 2, 3, 4];let arrayWithoutDuplicates = myArray.reduce(function (accumulator, element) {if (accumulator.indexOf(element) === -1) {accumulator.push(element);}return accumulator;}, []);console.log(arrayWithoutDuplicates); // [1, 2, 3, 4, 5]
Using the .reduce() method, you can efficiently and simply remove duplicate elements from an array. However, you should keep in mind that this method may be more complex to understand and use compared to other options.
3 - Removing Elements from an Array Using .forEach()
This method iterates through the array and executes a function for each element in the array.
To remove duplicate elements from an array using .forEach(), you need to create an empty object and add each element from the array to the object if it's not already in it. By the end of the process, the object will contain all the unique elements from the array.
let myArray = [1, 2, 3, 4, 5, 2, 3, 4];let uniqueObject = {};myArray.forEach(function (element) {uniqueObject[element] = element;});let uniqueArray = Object.values(uniqueObject);console.log(uniqueArray); // [1, 2, 3, 4, 5]
4 - Removing Duplicates from an Array Using the Set Object
This method creates an object that only allows unique values as elements.
To remove duplicate elements from an array using Set(), you need to create a new Set object from the array and then convert the Set object to an array using the Array.from() method.
let myArray = [1, 2, 3, 4, 5, 2, 3, 4];let uniqueSet = new Set(myArray);let uniqueArray = Array.from(uniqueSet);console.log(uniqueArray); // [1, 2, 3, 4, 5]
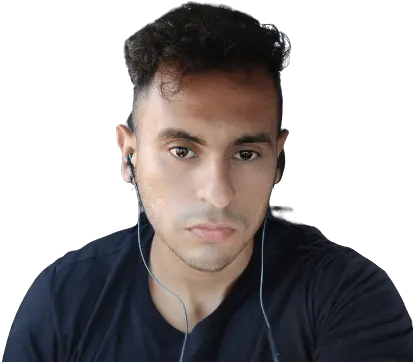
I am Juneiker Castillo, a passionate front-end web developer deeply in love with programming and creating fast, scalable, and modern websites—a JavaScript enthusiast and a React.js lover ⚛️.
About me