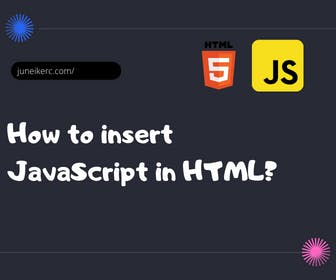
JavaScript is the widely dominant programming language for frontend development as it allows adding dynamic functionality to a website.
In this article, you will learn different ways to insert JavaScript into HTML.
Method 1: Using JavaScript in HTML from an External File
This is the most common way to execute JavaScript in HTML. Create a file and link it to your page using the script tag, for example:
<script src="script.js"></script>
Method 2: Including JavaScript in the Script Tag
You can place this tag anywhere in your HTML document, but it's more common to put it at the end of the document, just before the closing body tag.
For example, if you want to insert an alert message on your web page, you can use the following code:
<script>alert("Hello world");</script>
Method 3: Including JavaScript in an Event Attribute
Another way to insert JavaScript into HTML is by including it in an event attribute. Event attributes are special attributes that get triggered when a specific event occurs on the web page, such as clicking a button or loading the page.
For example, if you want to display an alert message when the user clicks a button, you can use the following code:
<button onclick="alert('Hello, world')">Click here</button>
It's also possible to include a JavaScript function instead of a simple statement. For example:
<button onclick="myFunction()">Click here</button><script>function myFunction() {alert("Hello, world");}</script>
Method 4: Including JavaScript in a Template Tag
Template tags are special tags used to store content that is not immediately displayed on the web page.
To include JavaScript in a template tag, you should enclose the JavaScript code between the template tags. Then, you can use the .innerHTML method to insert the content from the template tag into the HTML document.
For example, if you want to insert an alert message on your web page using a template tag, you can use the following code:
<template id="myTemplate"><script>alert('Hello, world');</script></template>
let template = document.getElementById("myTemplate");document.body.innerHTML = template.innerHTML;
It's important to note that template tags are not automatically processed by the browser. You must use JavaScript to insert the content of the template tag into the HTML document.
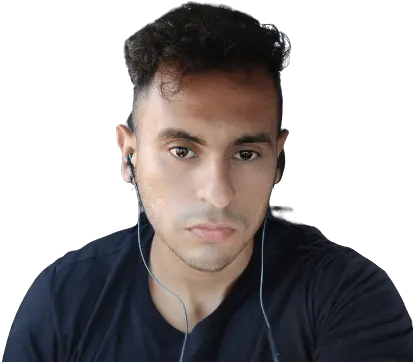
I am Juneiker Castillo, a passionate front-end web developer deeply in love with programming and creating fast, scalable, and modern websites—a JavaScript enthusiast and a React.js lover ⚛️.
About me