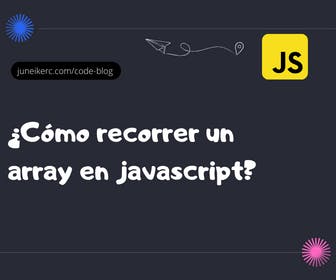
Iterating through an array is one of the tasks we frequently have to do in the world of web development, as arrays are by far the most commonly used data structure in programming. In this tutorial, you will learn how to iterate through them with JavaScript.
Iterating through an array with the for loop
const arr = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
for (let i = 0; i < arr.length; i++) {console.log(arr[i]);}//1//2//3//4//5//...
Very easy, right? We wrote a for loop that prints the position of each of the values within the array.
Now, let's move on to a more advanced example. We're going to create a function that iterates through the array and returns only the elements in the array that are greater than 10.
function greaterThanTen(arr) {let newArray = [];for (let i = 0; i < arr.length; i++) {if (arr[i] > 10) {newArray.push(arr[i]);}}return newArray;}greaterThanTen([2, 12, 8, 14, 80, 0, 1]);// returns [12, 14, 80]
The conditional within the loop instructs it to add any number greater than 10 to the new array. The for loop is the most versatile for working with arrays, but there are other options that make the task much easier and more intuitive.
Iterating through an array using forEach in JavaScript
The forEach method allows us to iterate through an array much more easily than the for loop. forEach() executes a callback for each item within the array and iterates through them in ascending order.
const arr = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];arr.forEach(element => {console.log(element);});//1//2//3//4//...
In this case, we iterate through the array of numbers using forEach(), and for each element, we use console.log() to print the respective element. Please note that this is a very basic exercise, but you can implement this method in much more advanced scenarios.
Iterating through an array of objects with JavaScript
const arrObj = [{name: "John",lastName: "Doe",},{name: "Margarita",lastName: "Gonzales",},{name: "Manuel",lastName: "Linares",},];
This is very simple, so let's go for it.
for (let i = 0; i < arrObj.length; i++) {console.log(arrObj[i].lastName);}//Doe//Gonzales//Linares
Iterating through a two-dimensional array with JavaScript
First, let's define the matrix we're going to iterate over. You can iterate through an array within another array with JavaScript as follows:
const biArray = [[1, 2, 3],[4, 5, 6],[7, 8, 9],];for (let i = 0; i < biArray.length; i++) {for (let j = 0; j < biArray.length; j++) {console.log(biArray[i][j]);}}//1//2//3//....
And even easier.
biArray.forEach(element => {element.forEach(elem => {console.log(elem);});});//1//2//3//....
Iterating Using .map()
The .map() method iterates through each item in an array and returns a new array containing elements specified in the callback. It does all of this without changing the original array. Let's look at an example where we extract elements from an array of objects.
const users = [{name: "John",lastName: "Doe",},{name: "Margarita",lastName: "Gonzales",},{name: "Manuel",lastName: "Linares",},];const names = users.map(user => user.name);console.log(names);// [ 'John', 'Margarita', 'Manuel' ]
Well, you now have different ways to iterate through an array in JavaScript. I hope I've been able to help, and thank you for reading.
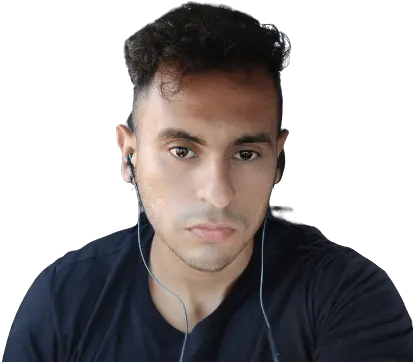
I am Juneiker Castillo, a passionate front-end web developer deeply in love with programming and creating fast, scalable, and modern websites—a JavaScript enthusiast and a React.js lover ⚛️.
About me