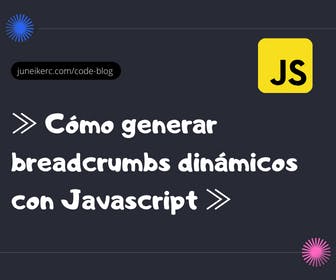
Efficient navigation is essential for any website. When users visit your page, they want to know where they are and how they can easily go back or explore other sections.
Breadcrumbs are an effective way to achieve this. In this article, we will explore JavaScript code that allows you to dynamically generate breadcrumbs and enhance the user experience on your website.
What are breadcrumbs?
Breadcrumbs are a series of navigation links that indicate the path a user has followed to reach a specific web page.
They are typically displayed at the top of the page and enable users to easily return to previous pages.
Imagine you have a website with multiple categories and subcategories. When a user navigates deeply into your site and then decides to go back to the homepage or a higher-level category, breadcrumbs provide a clear path to do so.
This not only enhances the user experience but also simplifies navigation and understanding of your site's structure. This can contribute to improving the SEO (Search Engine Optimization) of your website.
Code to Generate Dynamic Breadcrumbs from a URL with JavaScript
const generateBreadcrumbs = (pathname) => {const pathNames = pathname.split("/").filter((path) => path);const breadcrumbs = pathNames.map((item, i) => {const href = `/${pathNames.slice(0, i + 1).join("/")}`;const anchor = `${item.replaceAll("-", " ")}`;return {href,anchor,};});breadcrumbs.unshift({ href: "/", anchor: "Home" });return breadcrumbs;};/*console.log(generateBreadcrumbs('/nike/shoes/sports/red'))[{ href: '/', anchor: 'Home' },{ href: '/nike', anchor: 'nike' },{ href: '/nike/shoes', anchor: 'shoes' },{ href: '/nike/shoes/sports', anchor: 'sports' },{ href: '/nike/shoes/sports/red', anchor: 'red' }]*/
This code is a function called generateBreadcrumbs that takes a pathname string as input and returns a set of breadcrumbs in the form of an array of objects. Now, let's break down how this code works.
- Path Splitting: The function starts by splitting the pathname into fragments using split("/"). Then, it filters out empty fragments to ensure there are no unwanted elements in the path.
- Generating Links and Anchors: It then iterates through the path fragments and generates both the link (href) and anchor text (anchor) for each fragment. Fragments are joined to create the link's URL, and hyphens are replaced with spaces in the anchor text to make it more readable.
- Adding a Home Page: Finally, an additional breadcrumb is added to the beginning of the array, which typically represents the website's home page.
Implementation on Your Website
Now that you have the breadcrumb generation code, it's time to implement it on your website. Here's a step-by-step guide on how to do it:
Step 1: Import the Function
Make sure to import the generateBreadcrumbs function in the file where you want to display the breadcrumbs.
import { generateBreadCrumbs } from './breadcrumbs.js';
Step 2: Get the Current Path
Before using the function, you need to obtain the current page's path. You can do this using window.location.pathname or any equivalent method in your development environment.
Step 3: Generate the Breadcrumbs
Call the generateBreadcrumbs function with the current path as an argument to generate the breadcrumbs. This will give you an array of objects representing each path fragment.
const currentPath = window.location.pathname;const breadcrumbs = generateBreadCrumbs(currentPath);
Step 4: Display the Breadcrumbs
Finally, display the breadcrumbs on your website, preferably at the top. You can use HTML and CSS to style them according to your site's design.
Benefits of Breadcrumbs
Now that you have successfully implemented breadcrumbs on your website, it's essential to understand the benefits they bring:
- Intuitive Navigation: Users can navigate your site more intuitively and understand its structure.
- Improved User Experience: Breadcrumbs make it easy to return to previous pages, enhancing the user experience.
- Better SEO: Search engines value clear navigation, which can improve your position in search results.
- Reduced Bounce Rate: By providing simpler navigation, users are more likely to stay on your site for a longer time.
If you have successfully implemented breadcrumbs on your website or have any additional tips, share them in the comments section! Together, we can continue to improve the web browsing experience.
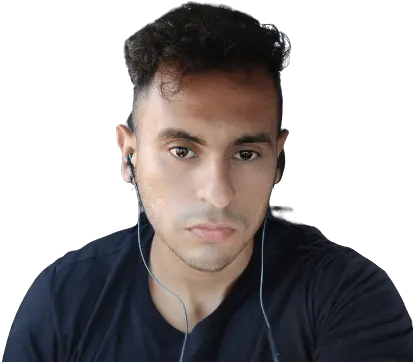
I am Juneiker Castillo, a passionate front-end web developer deeply in love with programming and creating fast, scalable, and modern websites—a JavaScript enthusiast and a React.js lover ⚛️.
About me