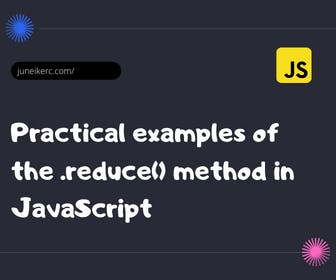
Do you know what the .reduce() method is and what it's used for? In this article, I'll explain how you can use this method in JavaScript to perform operations on array elements and obtain a single value as a result.
Additionally, I'll show you 6 practical and real-world examples of how you can apply the .reduce() method in different situations.
What is the .reduce() method and how does it work?
The .reduce() method is a function that belongs to the array prototype in JavaScript. This means that we can invoke it on any array, passing a reducing function as an argument, which will be executed on each element of the array.
The reducing function receives four parameters:
- Accumulator: This is the value that accumulates in each iteration of the reducing function. Ultimately, this will be the value returned by the .reduce() method.
- Current element: This is the element of the array being processed in each iteration.
- Current index: This is the index of the current element in the array.
- Original array: This is the array on which the .reduce() method is invoked.
The reducing function should return a value that will be assigned to the accumulator in the next iteration. The initial value of the accumulator can be specified as the second argument of the .reduce() method.
If not specified, the initial value will be the first element of the array, and the reducing function will start executing from the second element.
The syntax of the .reduce() method is as follows:
array.reduce((accumulator, currentElement, currentIndex, originalArray) => {// Reducing function logicreturn newAccumulatorValue;}, initialAccumulatorValue);
Example 1: Sum of Array Elements with JavaScript:
Let's look at a simple example of how to use the .reduce() method to sum all the elements of a numeric array:
// Create an array of numbersconst numbers = [1, 2, 3, 4, 5];// Use the .reduce() method to sum themconst sum = numbers.reduce((accumulator, currentValue) => {// Return the sum of the accumulator and the current valuereturn accumulator + currentValue;});// Display the result in the consoleconsole.log(sum); // 15
- In this case, the reducing function takes two parameters: the accumulator and the current value.
- Since we haven't specified an initial value, the accumulator takes the first element of the array (1) as the initial value, and the current value takes the second element of the array (2) as the initial value.
- The function returns the sum of both values (3), which becomes the new value of the accumulator. Then, the function runs again with the new accumulator value (3) and the next element of the array (3), returning the sum of both values (6), and so on until it iterates through the entire array and obtains the final sum (15).
Example 2: Concatenating Arrays with JavaScript:
const arrays = [[1, 2, 3],[4, 5, 6],[7, 8, 9],];const unifiedArray = arrays.reduce((acc, cur) => acc.concat(cur), []);console.log(unifiedArray); // [1, 2, 3, 4, 5, 6, 7, 8, 9]
In this example, we have an array of arrays called arrays, and we want to obtain a single array containing all the elements from the inner arrays.
To achieve this, we use the .reduce() method with a reducing function that takes two parameters: acc and cur. The acc parameter represents the accumulated array up to that point, and the cur parameter represents the current array from the main array.
The reducing function uses the .concat() method to concatenate the acc array with the cur array and returns the resulting new array. The second argument of the .reduce() method is [], which is used as the initial value for acc. Ultimately, the .reduce() method returns the final array from acc, which contains all the elements from the inner arrays.
const arrays = [["A", "B", "C"],["D", "E", "F"],["G", "H", "I"],];const unifiedArray = arrays.reduce((acc, cur) => acc.concat(cur), []);console.log(unifiedArray); // ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I']
In this another example, we have an array of arrays called arrays, and we want to obtain a single array containing all the letters from the inner arrays.
The procedure is the same as in the previous example, only the name of the array and the variable that stores the result change.
Example 3: Calculate the Average of Values within an Array
const lastGamePoints = [110, 102, 95, 98, 123, 145, 99];const averagePointsPerGame = lastGamePoints.reduce((acc, curr) => acc + curr) / lastGamePoints.length;console.log(averagePointsPerGame); // 110.28571428571429
For this exercise, we have an array with the number of points scored in the basketball team's recent games, and we need to calculate the average points per game for the team.
To do this, we use the .reduce() method to calculate the total points and then divide it by the length of the original array, obtaining the value of the average points per game in the averagePointsPerGame constant.
Example 4: Calculate the frequency of an element appearing within an array in JavaScript
const words = ["cat","dog","mouse","elephant","tiger","lion","giraffe","snake","eagle","whale","dolphin","bear","penguin","koala","shark","crocodile","leopard","penguin","tiger","cat","snake","dolphin","lion","cat"];const frequency = words.reduce((acc, curr) => {acc[curr] = (acc[curr] || 0) + 1;return acc;}, {});console.log(frequency);/*{cat: 3,dog: 1,mouse: 1,elephant: 1,tiger: 2,lion: 2,giraffe: 1,snake: 2,eagle: 1,whale: 1,dolphin: 2,bear: 1,penguin: 2,koala: 1,shark: 1,crocodile: 1,leopard: 1}*/
For this example, we need to count how many times words repeat within an array.
- We have an array of words on which we must apply the .reduce() method.
- We create the constant "frequency" and apply the reduce method to the "words" array with parameters "acc" (accumulator) and "curr" (current value), and we set an empty object as the initial value.
- Inside the reducing function, we do this: "acc[curr] = (acc[curr] || 0) + 1". Remember that the accumulator starts as an empty object, so in each iteration, we are creating a property "acc[curr," which in this case is a word, and we assign the value "(acc[curr] || 0) + 1", meaning the value that the property already has or (0), and then we add 1.
- Then, we return the accumulator.
Example 5: Convert an Array of Objects into an Object
To convert an array of objects into an object, we can use the .reduce() method to create a new object that has the values of one of the properties of the objects in the array as properties. For example:
// Array of objects representing usersconst users = [{ id: 1, name: "Ana", age: 25 },{ id: 2, name: "Carlos", age: 32 },{ id: 3, name: "Elena", age: 28 },];// Reducing function that creates an object with users as propertiesconst convert = (object, user) => {// Use the user's id as the property name and assign the user as the valueobject[user.id] = user;// Return the updated objectreturn object;};// Invoke the .reduce() method on the array, passing the convert function and an empty object as the initial valueconst userObject = users.reduce(convert, {});// Display the result in the consoleconsole.log(userObject);/*{'1': { id: 1, name: 'Ana', age: 25 },'2': { id: 2, name: 'Carlos', age: 32 },'3': { id: 3, name: 'Elena', age: 28 }}*/
The .reduce() method is a powerful tool that can save you
In this article, we have explored what the .reduce() method is in JavaScript and how to use it in different practical cases with code examples.
The .reduce() method allows us to elegantly and concisely solve complex problems with arrays. However, we should also be careful to use it correctly and understand how it works.
Some tips for using the .reduce() method include:
- Always specify the initial value for the accumulator if you want to avoid errors or unexpected behaviors.
- Use descriptive names for the parameters of the reducing function and avoid modifying the original array or the accumulator directly.
- Use other array methods like .map(), .filter(), or .find() if you only want to transform, filter, or search for elements in the array since they are simpler and more expressive than .reduce().
- Combine the .reduce() method with other array methods or auxiliary functions to create more modular and reusable solutions.
I hope this article has been useful to you and has helped you better understand the .reduce() method in JavaScript.
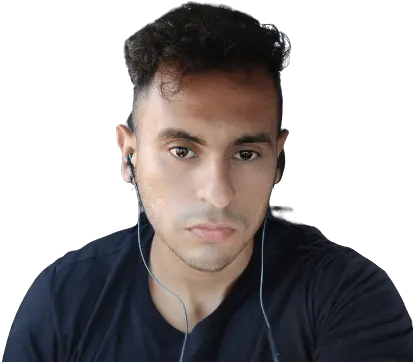
I am Juneiker Castillo, a passionate front-end web developer deeply in love with programming and creating fast, scalable, and modern websites—a JavaScript enthusiast and a React.js lover ⚛️.
About me