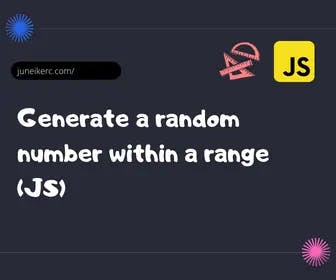
In JavaScript, thanks to the Math.random() function, we can generate a random number within a range of decimal values between 0 and 1, including 0 and excluding 1.
Math.random();// 0.45488628323782243
Generate a random number higher than 1 with js
Imagine the situation where you need to generate a random number below 100, to do this we must multiply the result of Math.random() by the number by which it must be below, in this case, 100.
Math.random() * 100;//21.93271361422726
As you can see, it still returns a decimal value. If you need an integer, we can round it down with the Math.floor() function.
Math.floor(Math.random() * 100);//21
Generate a random number within a range of two numbers min - max
We've reached the important part of this post, we need a minimum and a maximum value to write a function that returns a random value within a specified range.
"Equation" to solve this with javascript
- The first thing will be to create a function that must receive a minimum and a maximum value (min, max)
- We must generate a random number with Math.random()
- The generated number must be multiplied by the result of subtracting the minimum value from the maximum value
- To the result of the previous step, we add the value of the min parameter 5 Finally, we wrap the previous steps within the Math.floor() function to return an integer
Here you have the function randomNumberInRange expressed in JavaScript code for you to use in your project:
const randomNumberInRange = (min, max) =>Math.floor(Math.random() * (max - min)) + min;// randomNumberInRange(80,900) 583
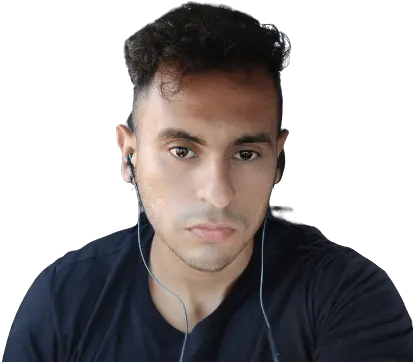
I am Juneiker Castillo, a passionate front-end web developer deeply in love with programming and creating fast, scalable, and modern websites—a JavaScript enthusiast and a React.js lover ⚛️.
About me