
There are many ways to center an image using CSS. In this tutorial, you will learn different methods to do it with just a few lines of code.
Centering an image in CSS without a container using display: block
Normally, when we work with images, we usually wrap them in a container element that we use to manipulate the position of the image.
Sometimes, we need to center images that are children of an element we can't manipulate, as it would affect the rest of the content. In such cases, we'll resort to the following trick (hack).
<img src="someImage.jpg" alt="css center image" />
By default, the img tag behaves like an inline element. If you want to align it to the center, simply set its display value to block and add an automatic margin to place it in the center, just as you would with any other element.
img {display: block;margin: auto;}
The above code will only center horizontally. Below, you will see how you can also center it vertically using grid and flexbox.
Centering an image both horizontally and vertically with CSS flexbox
For this example, we will center an image within a div using CSS flexbox.
<div class="div-img"><img src="source image" alt="center img with flexbox" /></div>
Code to center the image only horizontally
.div-img {display: flex;justify-content: center;}
Code to center the image within the div vertically
.div-img {display: flex;justify-content: center;align-items: center;}
Centering an image horizontally and vertically in HTML with CSS grid
Just like with flexbox, with grid, we can center elements using the `place-content` property. Let's center the same image from the previous div, but using this property.
<div class="photo-container"><img src="source image" alt="center photo" /></div>
.photo-container {display: grid;place-content: center;}
If you want to do it only horizontally, CSS grid, like flexbox, also has the justify-content property.
.photo-container {display: grid;justify-content: center;}
See an example of a centered image on CodePen.
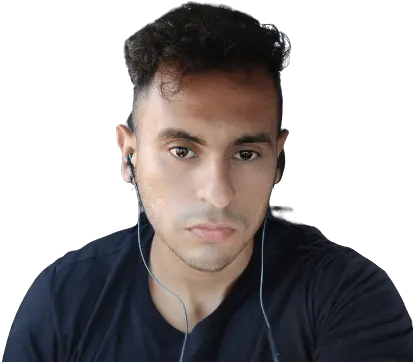
I am Juneiker Castillo, a passionate front-end web developer deeply in love with programming and creating fast, scalable, and modern websites—a JavaScript enthusiast and a React.js lover ⚛️.
About me