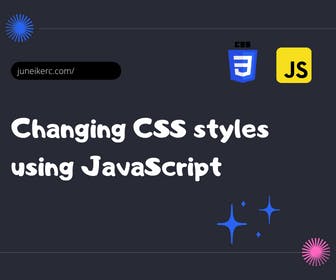
Modifying our CSS styles with JavaScript is a skill that every good web developer should master, and it's also very easy to achieve. So keep reading, and in the end, you'll see a quite entertaining exercise to practice.
Using the .style method in JavaScript, we can write new styles to an HTML tag.
Modifying CSS using .style with JavaScript
document.querySelector("button").style.background = "red";
In this case, we select the element with document.querySelector('button') and use .style to edit its background property and change it to red. In this particular case, it doesn't seem like a big deal, and honestly, this is quite useless, so let's do something more dynamic.
Let's define a basic HTML and CSS structure for this exercise.
<button id="buttonHorizontal">Horizontal</button><button id="buttonVertical">Vertical</button><div class="container"><div class="square"></div><div class="square"></div><div class="square"></div><div class "square"></div></div>
.container {max-width: 95%;margin: 0 auto;flex-wrap: wrap;}.square {width: 50px;height: 50px;background: teal;margin: 0.1em;}
Exercise to Change and Manipulate CSS Styles with JavaScript
What will we do in this exercise?
- We will change the background of our divs with the class "square." This background will be generated randomly each time we click on these elements.
- Change the axis of our "container" div depending on the button clicked.
function aleatorieColor() {const random1 = Math.ceil(Math.random() * 255);const random2 = Math.ceil(Math.random() * 255);const random3 = Math.ceil(Math.random() * 255);return `rgb(${random1},${random2},${random3})`;}document.querySelector("#buttonHorizontal").addEventListener("click", () => {document.querySelector(".container").style.display = "flex";document.querySelector(".container").style.flexDirection = "row";document.querySelector(".container").justifyContent = "space-around";});document.querySelector("#buttonVertical").addEventListener("click", () => {document.querySelector(".container").style.display = "flex";document.querySelector(".container").style.flexDirection = "column";document.querySelector(".container").justifyContent = "space-around";});document.querySelectorAll(".square").forEach(square => {square.addEventListener("click", e => {e.target.style.background = aleatorieColor();});});
- The randomColor function generates a random color in the RGB format, using Math.ceil(Math.random() * 255), which returns an integer between 0 and 255, the valid range for the RGB format.
- We select the buttons, and depending on which one is clicked, we will modify the orientation of the "container" by accessing the JavaScript .style method.
- Finally, using document.querySelectorAll('.square'), we get all elements with the class "square." Then, we iterate through them, and for each element, we execute a click event that modifies the background value to what the randomColor function returns.
NOTE: This way of modifying CSS from JavaScript does not change the actual file. What .style does is put the new styles inside the HTML tag, and in terms of specificity, these are the ones that are applied.
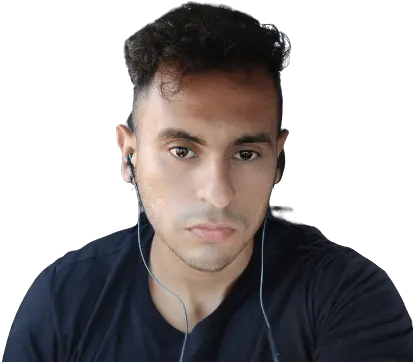
I am Juneiker Castillo, a passionate front-end web developer deeply in love with programming and creating fast, scalable, and modern websites—a JavaScript enthusiast and a React.js lover ⚛️.
About me