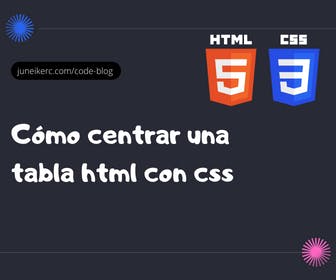
HTML tables allow us to present a lot of information in an organized way, so it's important to know how to work with them. Below, we present 3 ways to center an HTML table using CSS.
Centering an HTML Table with the 'margin: auto;' property
This is probably the most commonly used method to center an HTML table. Simply add 'auto' values to the margin-left and margin-right properties.
This is not the method I recommend, below you have two ways to do it with which you can have complete control over the position of the table within a container.
<table class="table"><tr><td><b>Name</b></td><td><b>Last Name</b></td><td><b>Date</b></td></tr><tr><td>Carlos</td><td>Castillo</td><td>02/11/1998</td></tr><tr><td>Pedro</td><td>Martínez</td><td>01/21/1990</td></tr></table>
.table {border: 1px solid black;margin-left: auto;margin-right: auto;}
<tablestyle="border:1px solid black;margin-left:auto;margin-right:auto;"></table>
Centering an HTML Table with Grid Display
Another option, and the one I personally recommend, is to use a layout property like grid or flexbox by wrapping the table you need in a container div.
That's what divs are for, to help us structure our content.
<div class="table-wrapper" ><table class="table" ><tr><td><b>Name</b></td><td><b>Last Name</b></td><td><b>Date</b></td></tr><tr><td>Carlos</td><td>Castillo</td><td >02/11/1998</td></tr><td>Pedro</td><td>Martínez</td><td >01/21/1990</td></tr></table></div>
.table-wrapper {display: grid;place-content: center;}.table {border: 1px solid black;}
Centering an HTML Table with Flex Display
<div class="table-wrapper" ><table class="table" ><tr><td><b>Name</b></td><td><b>Last Name</b></td><td><b>Date</b></td></tr><tr><td>Carlos</td><td>Castillo</td><td >02/11/1998</td></tr><td>Pedro</td><td>Martínez</td><td >01/21/1990</td></tr></table></div>
.table-wrapper {display: flex;justify-content: center;}.table {border: 1px solid black;}
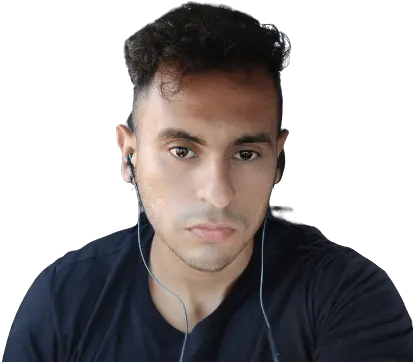
I am Juneiker Castillo, a passionate front-end web developer deeply in love with programming and creating fast, scalable, and modern websites—a JavaScript enthusiast and a React.js lover ⚛️.
About me