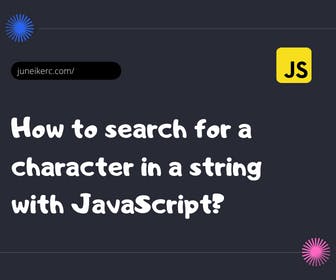
Sometimes solving certain programming problems can be overwhelming, but don't overcomplicate things. As you gain experience, these types of challenges will become easier for you.
Next, you will learn how to find the position of a character in a string using JavaScript in three different ways.
Finding the Position of a Character Using the .search() Method
The .search() method takes a regular expression or a string as a parameter and returns the index of the first match within the string. If no match is found, it returns -1.
const word = "some word";word.search("d"); // returns 8, index of the letter 'd'word.search("s"); // returns 0
Using the .indexOf() Method
The .indexOf() method can only be used on strings, and to search for characters, you need to pass a parameter with the letter you're looking for. It will return its index, and if it doesn't find it, it will return -1. Take a look at the following examples:
const word = "some word";word.indexOf("s"); // This returns 0word.indexOf("w"); // This returns 5 since the space is also counted as a character
Using the .includes() Method
Although technically it doesn't find the position of a character, this method is used to check whether the string contains the text or not, and it will return true or false. Look at the following examples:
const word = "Any word";word.includes("A"); // true (if it were lowercase, the result would be false)word.includes("z"); // false// You can also pass wordsword.includes("word"); // trueword.includes("hello"); // false
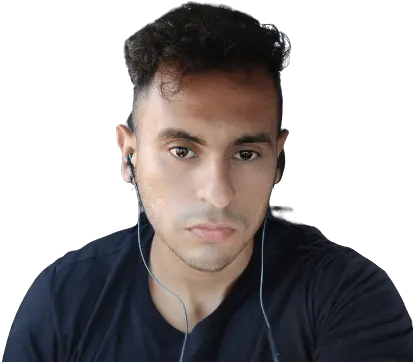
I am Juneiker Castillo, a passionate front-end web developer deeply in love with programming and creating fast, scalable, and modern websites—a JavaScript enthusiast and a React.js lover ⚛️.
About me