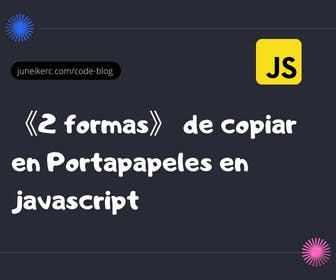
Have you ever wished to have a magical button that copies text to the clipboard on your website? You're in the right place! In this article, you will learn how to use JavaScript to copy text to the clipboard.
From how to use the basic functions to creating an HTML button that copies on click.
Using the asynchronous clipboard API navigator.clipboard
This API has been available for some time now, and if you don't need compatibility with older browser versions, this API is the easiest and safest way to copy content to the clipboard from the web.
const copyToClipboard = async str => {try {await navigator.clipboard.writeText(str);console.log("copied");} catch (error) {console.log(error);}};
The function called copyToClipboard takes a string str as an argument and uses the clipboard API (navigator.clipboard.writeText) to copy that string to the clipboard.
If the operation is successful, it will write the value of the str argument to the clipboard and print "copied" to the console. If there is any error, it will log it to the console.
Note that this code assumes you are running it in an environment that supports the clipboard API, such as a modern web browser.
In some environments (for example, server-side environments), the clipboard API may not be available, so you should be cautious when using this function in different contexts like in applications of Next.js, Remix, Gatsby, or Qwik.
Example of copying to the clipboard in JavaScript through a button
One way to implement the previous function to copy the string to the clipboard is to listen for a click event and register a value in the clipboard.
The value to copy can be the text of an element or the value of an input, so when the click event is triggered, the element will also be copied to the clipboard. Let's see an example:
<blockquote id="quote">Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod temporincididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quisnostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.</blockquote><button id="buttonCopy">copy</button>
const button = document.getElementById("buttonCopy");const quote = document.getElementById("quote");const copyToClipboard = async str => {try {await navigator.clipboard.writeText(str);console.log("copied");} catch (error) {console.log(error);}};button.addEventListener("click", () => {copyToClipboard(quote.textContent);});
Copy to Clipboard with Document.execCommand('copy')
In case the previous option is not suitable for you, either because your target audience frequently uses older browsers or you don't understand the previous implementation. You can use Document.execCommand('copy') to achieve the same result.
Step by step to copy to the clipboard with Document.execCommand from JavaScript
- Create a textarea element to add to the document.
- Add the value you want to copy.
- Add the textarea to the current HTML document and use CSS to hide it to prevent flickering.
- Use HTMLInputElement.select() to select the content of the textarea.
- Now, with Document.execCommand('copy'), we can copy the content of the textarea to the clipboard.
- Remove the textarea from the document.
const copyToClipboard = str => {const textArea = document.createElement("textarea");textArea.value = str;// Add the element to the DOMdocument.body.appendChild(textArea);// Select the text in the textareatextArea.select();try {// Copy the text to the clipboarddocument.execCommand("copy");console.log("Copied to clipboard");} catch (err) {console.error("Could not copy to clipboard:", err);}// Remove the textarea from the DOMdocument.body.removeChild(textArea);};
Implementation of a button to copy text to the clipboard using the execCommand method
<blockquote id="quote">consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore etdolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitationullamco laboris nisi ut aliquip ex ea commodo consequat.</blockquote><button id="buttonCopy">copy</button>
const button = document.getElementById("buttonCopy");const quote = document.getElementById("quote");function copyToClipboard(text) {const textArea = document.createElement("textarea");textArea.value = text;// Add the element to the DOMdocument.body.appendChild(textArea);// Select the text in the textareatextArea.select();try {// Copy the text to the clipboarddocument.execCommand("copy");console.log("Copied to clipboard");} catch (err) {console.error("Could not copy to clipboard:", err);}// Remove the textarea from the DOMdocument.body.removeChild(textArea);}button.addEventListener("click", () => {copyToClipboard(quote.textContent);});
You should keep in mind that this will only respond to a user action (for example, on a button to copy text) due to internal workings of this method.
See both examples in action.
Experiment with these techniques and add the ability to copy to the clipboard in your web projects to make them even more useful and user-friendly. Your visitors will appreciate it!
If you found this article helpful, share it with other developers so they can also take advantage of this valuable JavaScript feature!
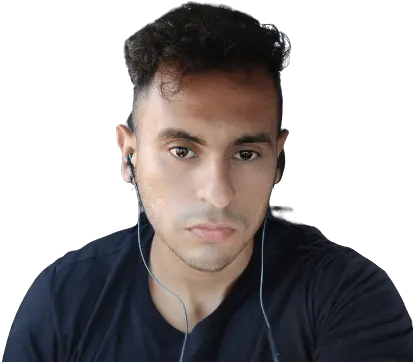
I am Juneiker Castillo, a passionate front-end web developer deeply in love with programming and creating fast, scalable, and modern websites—a JavaScript enthusiast and a React.js lover ⚛️.
About me