What is Next.js? The React framework for the web
Would you like to create dynamic, interactive, and fast web applications with React? Would you like to have a framework that makes development and project configuration easier for you? Do you want to take advantage of the latest React features, such as Server Components and Actions? Next.js is the framework you are looking for.
In this article, we will explain what Next.js is, its main features, how to install it, and how to get started using it.
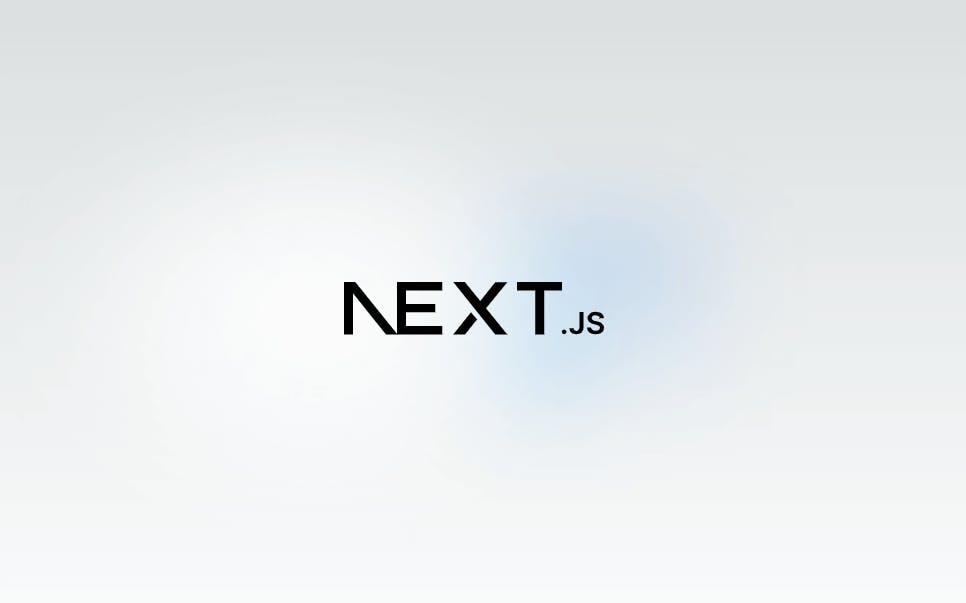
What is Next.js? and What is its Relationship with React.js?
Next.js is a server-side rendering framework for React that enables you to create full-stack web applications with the latest features and optimizations of React. While built upon React, Next.js adds additional functionalities that make development easier and results in faster outcomes.
Next.js automatically abstracts and configures the necessary tools for React, including packaging, compilation, routing, rendering, data fetching, style support, optimizations, and more. This allows you to focus on building your application instead of dealing with configuration details.
Moreover, Next.js allows you to leverage the latest advancements in React, such as Server Components and Actions, enabling the creation of more dynamic, customized, and scalable web applications by harnessing the power of the server and edge.
Next.js is compatible with existing React applications, allowing you to migrate your project to Next.js without rewriting all the code. You can also use Next.js in conjunction with other React libraries such as Redux, Apollo, Material-UI, etc.
What are the main features of Next.js?
Next.js boasts numerous features that make it a comprehensive and powerful React framework. Some of the key features include:
- Routing: Next.js has a file-based routing system, allowing you to create routes using the folder and file structure of your project. It supports dynamic, nested, parameterized, lazy-loaded, loading state, error handling routes, and more.
- Rendering: Next.js enables server-side rendering (SSR) of applications, utilizing both client components and server components to enhance the user experience by delivering less JavaScript to the browser. Additionally, Next.js provides flexible rendering and caching options, such as Incremental Static Regeneration (ISR), on a per-page basis. Next.js also allows streaming the user interface from the server, integrated with the app router and React Suspense.
- Data Fetching: Next.js simplifies data fetching with async/await in Server Components and an extended fetch API for request memoization, data caching, and revalidation. It supports data fetching on both the server and the client.
- Style Support: Next.js allows you to style your application using your preferred methods, including CSS Modules, Sass, Tailwind CSS, styled-jsx, and more. It also supports global CSS and CSS variables.
- Optimizations: Next.js provides automatic optimizations for images, fonts, and scripts to enhance user experience and Core Web Vitals metrics.
- TypeScript: Next.js offers improved support for TypeScript, with better type checking and compilation, as well as a custom TypeScript plugin and type checker.
- Route Handlers: Next.js enables the creation of API endpoints for secure connections to third-party services, allowing consumption from your frontend.
- Middleware: Next.js allows you to take control of incoming requests, using code to define routing and access rules for authentication, experimentation, and internationalization.
- Server Components: Next.js lets you add components without sending additional JavaScript to the client, built on the latest React features.
- Actions: Next.js enables the creation of server-side actions that can be invoked from the client. Actions are functions that receive a request object and return a response object, utilizing your application's data and logic.
- SSR and SSG: It also allows you to choose between the types of sites you want to generate for your users, from highly dynamic sites to completely static pages.
What are the advantages of using Next.js?
Using Next.js provides numerous advantages for both developers and end-users. Some of the advantages of using Next.js include:
- Improved SEO: Next.js simplifies search engine optimization by providing server-side rendering (SSR) by default. This enhances your application's visibility on search engines, as the content is indexable from the server.
- Ease of Use: Next.js streamlines development and project configuration by providing a structure and convention for organizing your code, automatically configuring and optimizing the necessary tools for React. With Next.js, you can create a complete web application with React without worrying about technical details.
- Gradual Migration: If you already have an existing React-based application, Next.js facilitates a gradual migration. You can integrate Next.js into specific sections of your application without rebuilding the entire project.
- Performance: Next.js offers various rendering and caching options, both on the client and server sides, allowing you to optimize the performance and generation of your application. Next.js also includes built-in optimizations to improve the user experience and Core Web Vitals, such as image, font, and script optimization.
- Flexibility: Next.js allows you to choose from different styling options, data fetching methods, route handlers, and middleware based on your needs and preferences. It also enables you to use the latest React features, such as server components and actions, and leverage the fast, production-quality tools used by Next.js.
- Scalability: Next.js enables the creation of full-stack web applications with React and Node.js, easily scalable with edge and serverless functions. Next.js also allows you to create API endpoints to securely connect with third-party services and consume them from your frontend.
- Active Community and Ecosystem: Next.js has an active community and a robust ecosystem. There is a wide range of plugins, libraries, and resources available that can accelerate development and solve common problems.
Considerations and Disadvantages:
- Initial Learning Curve: While Next.js simplifies many aspects of development, there may be an initial learning curve for those unfamiliar with its concepts and features.
- Complexity for Small Projects: For small and simple projects, adopting Next.js may seem excessive and add unnecessary complexity. In such cases, a lighter configuration might be more suitable.
- Custom Configuration: Although default configuration is highly useful, in some cases, the need for more advanced custom configuration may pose a challenge.
- Possible Overhead for Static Projects: For projects that are primarily static and do not require dynamic features, the structure of Next.js may be more than necessary, affecting performance.
Remember that the choice to use Next.js or not will largely depend on the specific requirements of your project and the development team. Each tool has its strengths and weaknesses, and it's important to evaluate them based on your particular needs.
Are there examples of web applications created with Next.js?
Next.js is a highly popular React framework used by many companies and developers to create outstanding web applications with React. Some examples of web applications created with Next.js are:
- OpenAI and ChatGPT: OpenAI, a leading artificial intelligence organization, uses Next.js in the implementation of web applications, such as the ChatGPT user interface. Next.js enables OpenAI to create interactive and efficient browser experiences.
- Vercel: Vercel is the company that created Next.js and offers a cloud platform for frontend development. Vercel uses Next.js to develop its own user interface, known for its modern and elegant design. The company also employs Next.js to optimize the performance and user experience of its application through static and dynamic rendering, UI streaming, and image optimization.
- Hulu: Hulu is a video streaming service offering movies, series, and TV shows. Hulu uses Next.js to create its user interface, adapting to different devices and resolutions. Hulu also leverages Next.js to optimize the performance and user experience of its web application.
Integration of Next.js and JAMStack
Next.js, as mentioned earlier, is a powerful React framework that facilitates the creation of full-stack sites with advanced features and optimizations. Now, let's explore how Next.js integrates with the JAMStack approach.
JAMStack, which stands for JavaScript, APIs, and Markup, is a modern web development architecture that focuses on separating the frontend and backend layers. It relies on pregenerating pages and delivering content through APIs, providing significant benefits in terms of performance and scalability.
Integration of Next.js and JAMStack Advantages:
- Improved Performance: The combination of Next.js and JAMStack allows leveraging the benefits of both static site generation (SSG) and dynamic page generation (SSR or ISR). This results in faster page loads and an enhanced user experience, as a significant portion of the content is pregenerated and cached.
- Scalability and Security: JAMStack facilitates scalability by separating the frontend and backend, enabling the delivery of static content through CDN services. This improves security by reducing potential vulnerabilities associated with the server.
- Easy API Implementation: With Next.js, you can easily create your APIs using the "route handlers" mentioned earlier. This integration facilitates connecting to external services and fetching data needed for your JAMStack application.
- Image and Resource Optimizations: Next.js provides automatic optimizations for images, fonts, and scripts, aligning perfectly with JAMStack's performance principles. This contributes to improving Core Web Vitals metrics, thereby enhancing SEO.
Examples of Next.js and JAMStack Implementation:
- Static Personal Blog: Utilizing Next.js static site generation (SSG), you can create a static personal blog where content is pregenerated during compilation and cached for quick delivery.
- Dynamic Online Store: Applying Next.js incremental static regeneration (ISR), you can develop an online store that displays real-time product information while still benefiting from pregeneration for efficient performance.
- Dynamic News Site: By integrating external APIs with Next.js route handlers, you can build a dynamic news site that automatically updates content based on the latest available information.
Considerations When Using Next.js with JAMStack:
- Decoupled Architecture: The integration of Next.js with JAMStack promotes a decoupled architecture, meaning you must consider the need for efficient communication between the frontend and backend through APIs.
- Selection of Backend Services: When adopting JAMStack, you should select backend services that are compatible with this architecture, such as API services, data storage, and serverless functions.
- Form and State Management: Since much of the content is managed on the frontend, effective strategies for handling forms and states should be chosen, such as using third-party services or serverless functions.
- Frontend and API Technology Proficiency: The development team must have expertise in both frontend technologies and API management, as JAMStack requires close collaboration between these two areas.
This integration with JAMStack offers a powerful and modern solution for web development, enabling the construction of fast, secure, and scalable applications with ease. The choice of this combination will depend on the specific requirements of your project and the preference and expertise of your development team.
How to Install Next.js and Get Started?
To install Next.js and get started, you need to have Node.js and npm installed on your machine.
To install Next.js, you can use the npx command, which allows you to run npm packages without installing them globally. To create a new Next.js application, open a terminal and run the following command:
npx create-next-app@latest
Follow all installation steps while keeping everything at default, meaning following the recommendations provided by the framework itself.
Need to install the following packages:Ok to proceed? (y) y✔ What is your project named? … my-next-app✔ Would you like to use TypeScript? … No / Yes✔ Would you like to use ESLint? … No / Yes✔ Would you like to use Tailwind CSS? … No / Yes✔ Would you like to use `src/` directory? … No / Yes✔ Would you like to use App Router? (recommended) … No / Yes✔ Would you like to customize the default import alias (@/*)? … No / Yes
This will create a folder named my-next-app and the necessary files for your project. It will also install the required dependencies, such as React and Next.js.
To start your Next.js application, navigate into the project folder and run the following command:
npm run dev
This will start a development server on port 3000. You can open your browser and navigate to http://localhost:3000 to see your application in action.
To begin using Next.js, you can edit existing files or create new files in the app folder. Each page.jsx file in this folder corresponds to a route in your application. For instance, the app/page.jsx.js file is rendered when you access the root route /, and the about/page.jsx file is rendered when you access the /about route.
To create routes in Next.js, each folder within the app folder should have a page.jsx file that exports a React component representing the page. You can use JSX to write your component in a declarative and readable way. Additionally, you can use any React components, whether they are your own or from third parties.
Here's an example of a page in Next.js exported from the app/page.jsx file:
import Image from "next/image";import styles from "./page.module.css";export default function Home() {return (<main className={styles.main}><div className={styles.center}><ImageclassName={styles.logo}src="/next.svg"alt="Next.js Logo"width={260}height={67}priority/></div></main>);}
This component will be rendered when you access the / route of your application. You can view the result in your browser and modify the code as you wish.
What are you waiting for to use Next.js?
With Next.js, you can create amazing web applications with React without worrying about technical details and take advantage of the benefits of an exceptional React framework.
If you enjoyed this article and found it helpful in learning what Next.js is and why it's an excellent React framework, I invite you to share it with your friends and contacts. This way, you can help them discover Next.js and create incredible web applications with React.
And if you need to hire a freelance Next.js developer to migrate or create a website with Next.js, feel free to contact me. I'll be happy to assist you. I have experience and expertise in Next.js and web development with React.
Don't wait any longer and start using Next.js today. You'll see how your web development with React becomes easier, faster, and more enjoyable. Next.js is the React framework for the web. What are you waiting for to give it a try?