Top 7 most used backend languages today
Web development is a highly dynamic and competitive field that requires staying up-to-date with the latest trends and technologies. One of the most important decisions you must make as a web developer is which backend language to use for your project.
The backend language is responsible for the logic, processing, and storage of data on the server, and it communicates with the frontend language.
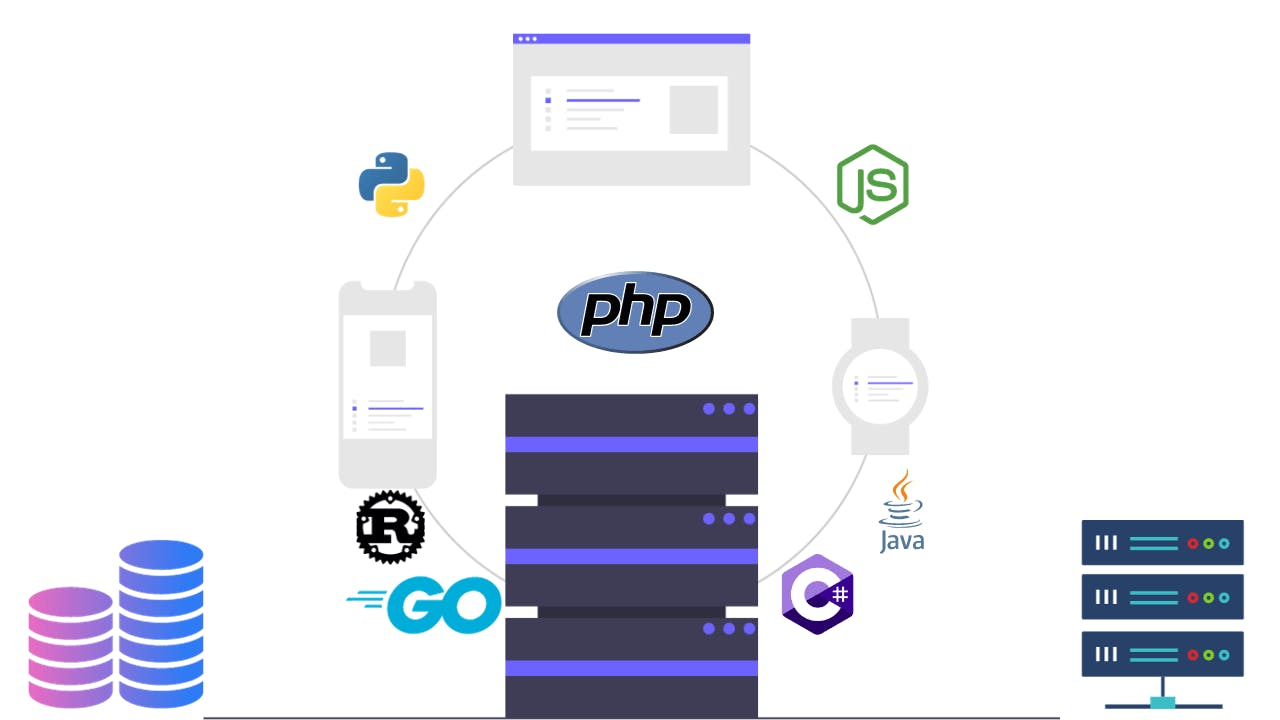
There are many backend languages available, each with its own characteristics, advantages, and disadvantages. Some are more suitable for certain types of projects than others, and some are easier to learn and use than others.
That's why, in this article, you have a list of 7 backend languages that we consider the best for a web project in 2023, along with their code examples and key virtues. This way, you can choose the one that best fits your needs and preferences.
1. Python: The most versatile and popular language
Python is one of the most popular and versatile languages globally, used for various applications, from data science, artificial intelligence, automation to web development.
Python has a simple and elegant syntax that makes code reading and writing easy. Additionally, it has a large developer community and a wide variety of libraries and frameworks that extend its functionalities.
One of the most used frameworks for web development with Python is Django, which provides a complete and robust environment with tools for authentication, security, administration, ORM, testing, and more.
Another highly popular framework is Flask, which is lighter and more flexible, allowing the quick creation of web applications with just a few lines of code.
Example of backend code using Python with the Flask framework:
# Import the Flask frameworkfrom flask import Flask, request, render_template# Create an instance of the applicationapp = Flask(__name__)# Define a route for the main page@app.route("/")def index():# Render an HTML templatereturn render_template("index.html")# Define a route for the form@app.route("/form", methods=["GET", "POST"])def form():# If the method is GET, display the formif request.method == "GET":return render_template("form.html")# If the method is POST, process the form dataelse:# Get the form dataname = request.form.get("name")email = request.form.get("email")message = request.form.get("message")# Do something with the data, for example, save it to a database# ...# Display a thank you messagereturn render_template("thankyou.html", name=name)
Use Cases of Python:
- Python is used to create various types of web applications, from blogs, social networks, educational platforms to e-commerce, data analysis, artificial intelligence, and more. Some examples of famous websites using Python include Google, YouTube, Instagram, Netflix, Spotify, Reddit, Quora, etc.
- Python is also used for creating desktop applications, mobile apps, games, scientific software, and more.
Key Virtues of Python:
- Python is an easy-to-learn and use language with clear and concise syntax, promoting productivity and code readability.
- Python is a multiparadigm language, allowing the use of different programming styles such as imperative, object-oriented, functional, procedural, etc.
- Python is an interpreted language that doesn't require prior compilation, enabling code execution on different platforms without modification.
- Python is a high-level language that abstracts many low-level details and provides a wide range of built-in functionalities, such as data structures, exceptions, generators, decorators, etc.
- Python is a dynamic language, allowing code modification at runtime, and it has a weak and dynamic typing system, facilitating flexibility and rapid development.
2. JavaScript: The Most Used Language in Frontend and Backend
JavaScript is the most widely used language in web development, both in frontend and backend. JavaScript is the only language that can be executed in the browser, enabling the creation of dynamic and interactive user interfaces.
Additionally, thanks to the Node.js platform, JavaScript can also run on the server and access resources such as files, databases, networks, etc.
Node.js is a JavaScript runtime environment based on the Google Chrome V8 engine, allowing the creation of scalable and high-performance web applications using the event-driven model and asynchronous programming paradigm.
Node.js also comes with a package manager called npm, offering thousands of modules and dependencies that can be easily installed and used.
Example code with Node.js:
// Import the http moduleconst http = require("http");// Create a serverconst server = http.createServer((req, res) => {// If the path is /if (req.url === "/") {// Send a response with the status code 200 (OK) and content type HTMLres.writeHead(200, { "Content-Type": "text/html" });// Send the content of the main pageres.end("<h1>Welcome to my website</h1>");}// If the path is /aboutelse if (req.url === "/about") {// Send a response with the status code 200 (OK) and content type HTMLres.writeHead(200, { "Content-Type": "text/html" });// Send the content of the about pageres.end("<h1>About me</h1><p>I am a web developer</p>");}// If the path is anything elseelse {// Send a response with the status code 404 (Not Found) and content type HTMLres.writeHead(404, { "Content-Type": "text/html" });// Send the content of the error pageres.end("<h1>Page not found</h1>");}});// Listen on port 3000server.listen(3000, () => {console.log("Server running on port 3000");});
Use Cases of JavaScript:
- JavaScript is used to create various types of web applications, from static and dynamic websites, single-page applications (SPA), progressive web apps (PWA), to real-time applications, streaming services, games, etc. Some examples of famous websites using JavaScript are: Facebook, Twitter, LinkedIn, Amazon, eBay, etc.
- JavaScript is also used to create desktop applications, mobile apps, hybrid apps, native apps, IoT applications, etc.
Key Virtues of JavaScript:
- JavaScript is a universal language, capable of execution in both the browser and the server, allowing the development of complete web applications with a single language.
- JavaScript is a flexible language, enabling the use of different programming styles, such as imperative, object-oriented, functional, reactive, etc.
- JavaScript is an interpreted language, eliminating the need for prior compilation and enabling code execution on different platforms without modification.
- JavaScript is a high-level language, abstracting many low-level details and providing a wealth of built-in functionalities, including data structures, regular expressions, promises, async/await, etc.
3. PHP: The Most Used Language on the Web
PHP is one of the most widely used languages in web development, running on the server-side and enabling the creation of dynamic and customized web applications. PHP has a syntax similar to C and can be easily embedded in HTML code.
Moreover, it boasts excellent compatibility with various operating systems, web servers, and databases.
One of the most used frameworks for backend development with PHP is Laravel, providing an elegant and expressive environment with tools for routing, validation, authentication, authorization, ORM, testing, and more.
Another highly popular framework is Symfony, known for its robust and professional nature, allowing the development of complex, large-scale web applications.
Example code with Laravel:
// Import the Laravel frameworkuse Illuminate\Support\Facades\Route;// Define a route for the main pageRoute::get("/", function () {// Return a viewreturn view("welcome");});// Define a route for the formRoute::post("/form", function () {// Get the form data$name = request("name");$email = request("email");$message = request("message");// Do something with the data, for example, save it to a database// ...// Return a view with the datareturn view("thankyou", ["name" => $name]);});
Use Cases of PHP:
- PHP is used to create various types of web applications, from blogs, forums, CMS, social networks, educational platforms to e-commerce, data analysis, etc. Some examples of famous websites using PHP are WordPress, Wikipedia, Facebook, Yahoo, etc.
Key Virtues of PHP:
- PHP is an easy-to-learn and use language, with a familiar and straightforward syntax that promotes rapid and simple development.
- PHP is a multiparadigm language, allowing the use of different programming styles such as imperative, object-oriented, functional, etc.
- PHP is an interpreted language, eliminating the need for prior compilation and enabling code execution on different platforms without modification.
4. Java: The Most Robust and Professional Language
Java is a programming language known for its robustness and professionalism, enabling the creation of high-quality, secure, and performant web applications.
Java has a syntax based on C and can be used both imperatively and in an object-oriented manner. Moreover, it offers a plethora of advanced features such as inheritance, polymorphism, abstraction, encapsulation, etc.
One of the most used frameworks for web development with Java is Spring, providing a comprehensive and modular environment with tools for routing, validation, authentication, authorization, ORM, testing, and more.
Another highly popular framework is Struts, which is simpler and more conventional, allowing the creation of web applications based on the MVC pattern.
Example code with Spring:
// Import the Spring frameworkimport org.springframework.boot.SpringApplication;import org.springframework.boot.autoconfigure.SpringBootApplication;import org.springframework.web.bind.annotation.GetMapping;import org.springframework.web.bind.annotation.PostMapping;import org.springframework.web.bind.annotation.RequestParam;import org.springframework.web.bind.annotation.RestController;// Annotate the class as a Spring Boot application@SpringBootApplication// Annotate the class as a web controller@RestControllerpublic class Application {// Define a main method to run the applicationpublic static void main(String[] args) {SpringApplication.run(Application.class, args);}// Define a route for the main page@GetMapping("/")public String index() {// Return a text stringreturn "Welcome to my website";}// Define a route for the form@PostMapping("/form")public String form(@RequestParam String name, @RequestParam String email, @RequestParam String message) {// Get the form data// Do something with the data, for example, save it to a database// ...// Return a text string with the datareturn "Thank you " + name + " for your message";}}
Key Virtues of Java:
- Java is a robust and professional language with a strict and consistent syntax, promoting code quality and security.
- Java is a multiparadigm language, allowing the use of different programming styles such as imperative, object-oriented, functional, concurrent, etc.
- Java is a compiled language, requiring prior compilation and generating an intermediate code called bytecode. This bytecode can be executed on different platforms without modification, thanks to the Java Virtual Machine (JVM).
- Java is a static language, featuring a strong and static typing system that facilitates error detection and prevention at compile time.
5. C#: The Most Powerful and Modern Language
C# is a programming language known for its power and modernity, enabling the creation of high-level web applications with features like object-oriented programming, generic programming, functional programming, concurrent programming, etc.
C# has a syntax based on C++, and it can be used both imperatively and in an object-oriented manner. Moreover, it offers a variety of advanced features, such as delegates, events, attributes, LINQ, async/await, etc.
One of the most used frameworks for web development with C# is ASP.NET, providing a comprehensive and efficient environment with tools for routing, validation, authentication, authorization, ORM, testing, and more.
Another highly popular framework is Blazor, allowing the creation of interactive web applications using C# instead of JavaScript, running either in the browser or on the server.
Example code with ASP.NET:
// Import the ASP.NET frameworkusing Microsoft.AspNetCore.Mvc;// Annotate the class as a web controller[Route("/")][ApiController]public class HomeController : ControllerBase{// Define a route for the main page[HttpGet]public IActionResult Index(){// Return a viewreturn View("Index");}// Define a route for the form[HttpPost("form")]public IActionResult Form(string name, string email, string message){// Get the form data// Do something with the data, for example, save it to a database// ...// Return a view with the datareturn View("ThankYou", name);}}
Key Advantages of C#:
- C# is a powerful and modern language with a clear and consistent syntax that promotes code efficiency and innovation.
- C# is a multiparadigm language, allowing the use of different programming styles such as imperative, object-oriented, generic, functional, concurrent, etc.
- C# is a compiled language, requiring prior compilation and generating intermediate code called IL (Intermediate Language), which can be executed on different platforms without modification, thanks to the .NET runtime environment.
- C# is a static language, featuring a strong and static typing system that facilitates error detection and prevention at compile time.
6. Go: The Fastest and Concurrent Language for Backend
Go is a programming language known for its speed and concurrency, enabling the creation of high-performance, scalable, and reliable web applications.
Go has a simple and clean syntax and can be used both imperatively and in an object-oriented manner.
Moreover, it offers a variety of advanced features, such as goroutines, channels, interfaces, slices, maps, etc.
One of the most used frameworks for backend development with Go is Gin, providing a lightweight and fast environment with tools for routing, validation, authentication, authorization, testing, and more.
Another highly popular framework is Revel, which is more comprehensive and productive, allowing the creation of web applications with the MVC pattern.
Example code with Gin:
// Import the Gin frameworkimport "github.com/gin-gonic/gin"// Create an instance of the applicationapp := gin.Default()// Define a route for the main pageapp.GET("/", func(c *gin.Context) {// Return a text stringc.String(200, "Welcome to my website")})// Define a route for the formapp.POST("/form", func(c *gin.Context) {// Get the form dataname := c.PostForm("name")email := c.PostForm("email")message := c.PostForm("message")// Do something with the data, for example, save it to a database// ...// Return a text string with the datac.String(200, "Thank you %s for your message", name)})// Listen on port 3000app.Run(":3000")
Key Virtues of Go:
- Go is a fast and concurrent language with a simple and clean syntax that promotes code efficiency and scalability.
- Go is a compiled language, requiring prior compilation and generating native binary code that can be executed on different platforms without modification.
- Go is a static language, featuring a strong and static typing system that facilitates error detection and prevention at compile time.
7. Rust: The Safest and Most Efficient Language
Rust is a programming language known for its safety and efficiency, enabling the creation of high-performance web applications without memory errors or bottlenecks.
Rust has a syntax inspired by C++, and it can be used both imperatively and in an object-oriented manner. Moreover, it offers a variety of advanced features such as ownership, borrowing, lifetimes, traits, macros, etc.
One of the most used frameworks for web development with Rust is Rocket, providing a comprehensive and productive environment with tools for routing, validation, authentication, authorization, ORM, testing, and more.
Another highly popular framework is Actix, which is lighter and faster, allowing the creation of web applications based on the actor model.
Example code with Rocket:
// Import the Rocket framework#[macro_use] extern crate rocket;// Define a route for the main page#[get("/")]fn index() -> &'static str {// Return a text string"Welcome to my website"}// Define a structure for the form data#[derive(FromForm)]struct FormData {name: String,email: String,message: String,}// Define a route for the form#[post("/form", data = "<form_data>")]fn form(form_data: FormData) -> String {// Get the form datalet name = form_data.name;let email = form_data.email;let message = form_data.message;// Do something with the data, for example, save it to a database// ...// Return a text string with the dataformat!("Thank you {} for your message", name)}// Create a main function to run the application#[launch]fn rocket() -> _ {// Start the application with the defined routesrocket::build().mount("/", routes![index, form])}
Choosing a Backend Language for Your Project
As you have seen, there are many backend languages available, each with its pros and cons, and some more suitable for certain types of projects than others.
Therefore, there is no single definitive answer to the question of which backend language to choose. It depends on various factors, such as:
- Your personal preferences and your level of experience as a web developer.
- The requirements and features of your web project, such as type, size, complexity, functionality, audience, etc.
- Available resources and tools, such as time, budget, team, server, framework, database, etc.
However, to help you make a more informed decision, we will provide some general recommendations based on the key virtues of each backend language discussed in this article:
- If you want a versatile and popular language that can be used for all types of web applications and has a large community with a wide variety of libraries and frameworks, we recommend Python.
- If you want a universal language that can be executed in the browser and on the server, allowing you to create complete web applications with a single language, we recommend JavaScript.
- If you want an easy and fast language that can be easily embedded in HTML code and has great compatibility with different operating systems, web servers, and databases, we recommend PHP.
I hope this article has been helpful and has provided you with a general overview of the best backend languages for your web project.
Remember that the final choice depends on you and your project, and the most important thing is that you feel comfortable and satisfied with the language you choose.